Rust has been one of the top 15 most popular and loved programming languages over the past five years due to the incredible developer experience it offers and its flexibility. It boasts powerful features, transparent documentation, and an engaged and supportive community of contributors.
Rust prioritizes safety, performance, and concurrency. It allows programmers to create applications with control of a low-level language, but with the powerful abstractions of a high-level language. Overall, there’s so much you can do with Rust that every developer should at least give it a try.
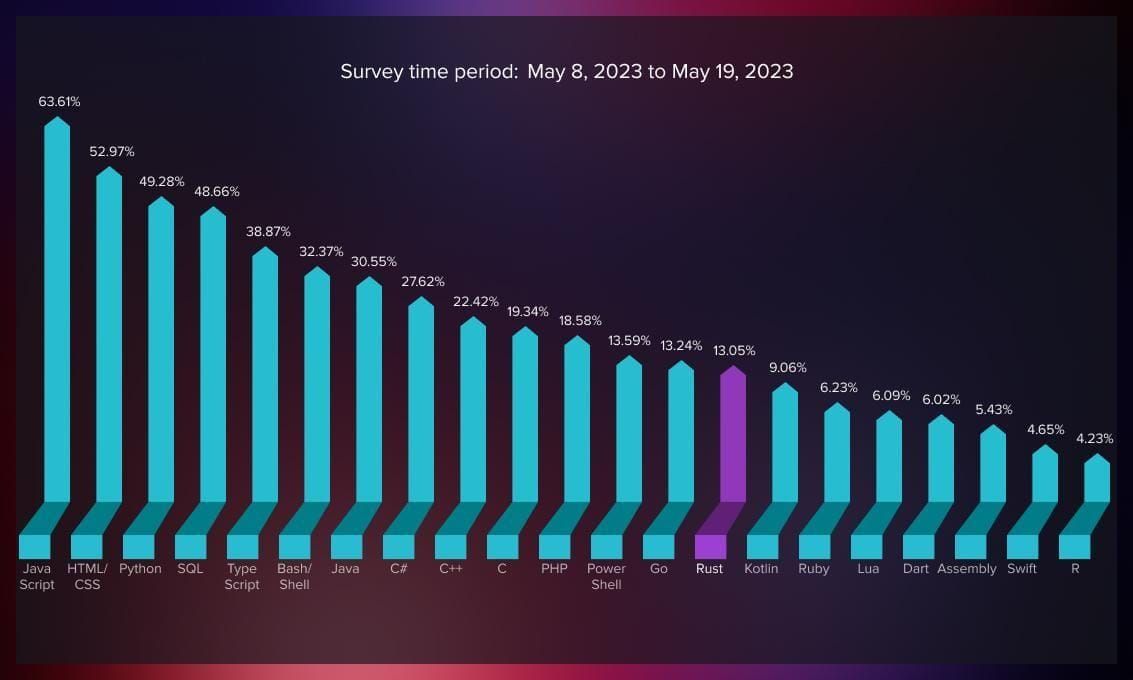
Source: Statista
What are the core Rust features?
Now let’s look at what makes Rust so effective and applicable to numerous use cases.
Memory safety
-
The primary design goal of Rust is to guarantee memory safety without relying on a garbage collector. This is achieved through transparent data ownership, a robust type system, and rigorous compile-time checks. Data ownership paradigm dictates that every piece of data in Rust has a unique owner. When ownership is transferred (known as a “move”), the previous owner can no longer access the data. This prevents duplicate free operations and eliminates double-deletion bugs.This approach eliminates a class of bugs right at the compile stage.
-
Bugs like null pointer dereferencing, which is a prevalent issue in many languages, are eliminated by using the
Option<T>
type in Rust. It explicitly handles the possibility of absence of value. -
Data races and buffer overflows are also prevented due to Rust’s borrowing and ownership system. If a piece of code is potentially unsafe, Rust won’t compile it unless wrapped in an
unsafe
block, signaling the developer to proceed carefully.
Concurrency model
-
Concurrency in Rust is built upon the same principles that offer memory safety: ownership and borrowing. By leveraging these, Rust ensures thread safety.
-
The language offers several concurrency primitives, like
Arc
(atomic reference counting) for shared state andMutex
orRwLock
for mutable access. Channels in Rust offer a way to communicate between threads. -
The
async/await
syntax, introduced in Rust 1.39.0, allows for writing asynchronous code that looks like synchronous code, simplifying complex concurrency scenarios.
Performance characteristics
-
Rust’s “zero-cost abstractions” mean that using high-level constructs doesn’t impose a runtime overhead. You get the benefit of abstraction without sacrificing performance.
-
Rust, being a systems language, provides extensive control over hardware resources. This makes it as performant as C and C++ in many benchmarks, but with added safety guarantees.
-
Inlining, loop unrolling, and other aggressive optimizations can be performed due to Rust’s explicitness and lack of a runtime.
Watch this comprehensive video to learn more about Rust features:
The above features aren’t unique to Rust; its strength lies in their effective combination. Many Rust properties are inspired by or borrowed from other languages and various models:
-
Core foundations:
- Abstract machine model: Inspired by C.
- Memory model and management: Borrowed from languages and tools like C++, ML Kit, and Cyclone.
-
Data structures & types:
- Data types: Rust’s data types have roots in C, SML, OCaml, Lisp, and Limbo.
- Optional bindings: This feature is influenced by Swift.
-
Programming paradigms:
- Functional programming: Elements taken from Haskell, OCaml, and F#.
- Hygienic Macros: Adapted from Scheme.
-
Advanced features:
- Attributes: Deriving from the ECMA-335 standard.
-
Packaging & modularity:
- Crate system: Analogous to the Assembly in the ECMA-335 CLI model.
-
Concurrency & communication:
- Channels and concurrency: Influences from Newsqueak, Alef, and Limbo.
- Message passing and thread failure: Principles borrowed from Erlang.
Why adopt Rust development?
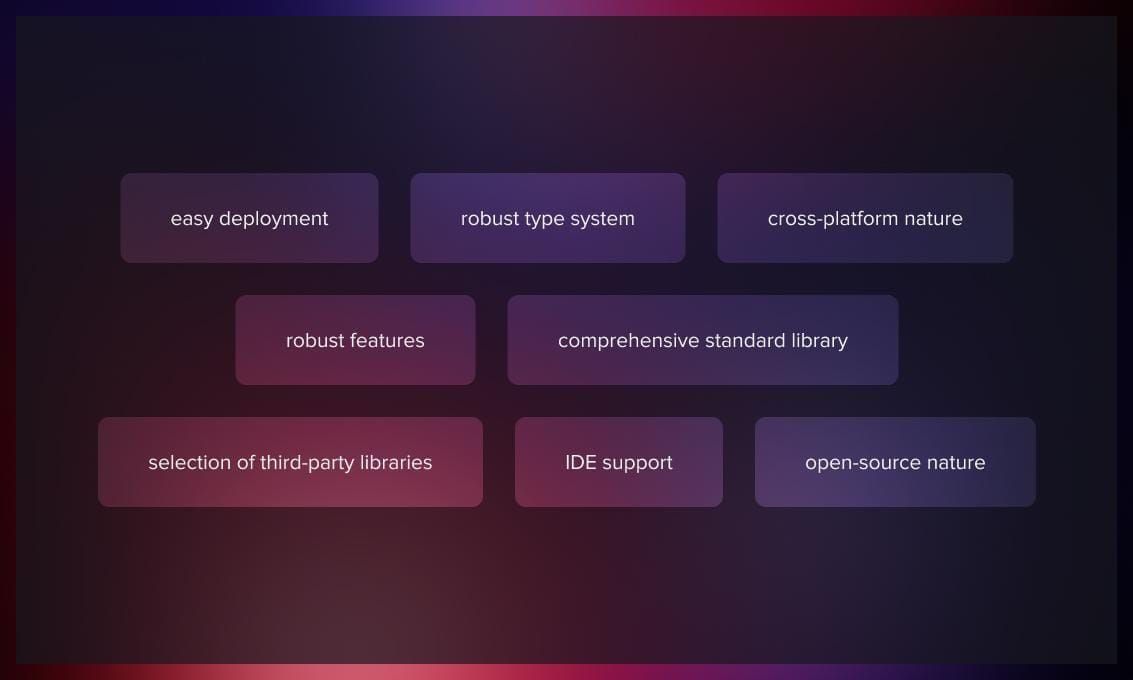
Rust combines various programming styles: procedural, actor-based concurrency, object-oriented, and purely functional. It also supports both static and dynamic forms of generic and metaprogramming. Below we provide an overview of the main Rust benefits.
Easy deployment
Rust’s robust safety and integrity tools wouldn’t mean much without active utilization. Rust’s creators and community make the language accessible and friendly for beginners. The package required to generate Rust binaries is unified. You need external compilers like GCCO only when working with components outside of the Rust system, like compiling a C library from its source.
Robust type system
With Rust, you aren’t allowed to compile code that contains detectable bugs. This feature enables developers to concentrate on the program’s core logic rather than on rectifying issues.
Cross-platform nature
While Rust is compatible with most modern platforms, its developers don’t aim to make it universal, focusing on popular platforms. Rust functions on three major operating systems: Linux, Windows, and MacOS. If there’s a need to cross-compile or generate binaries for another platform or architecture, Rust makes it relatively easy as well.
Robust features
Rust boasts a set of native features that can rival those in C++: Macros, generics, pattern matching, and composition via “traits.”
Comprehensive standard library
Part of Rust’s broader objective is to present a compelling alternative to C and C++. As such, it offers an extensive standard library that includes containers, collections, iterators, string operations, process and thread management, and more. Since Rust aims to be cross-platform, its standard library only includes universally portable features. Platform-specific functions are available through third-party libraries. Moreover, Rust can function without its standard library, which is especially useful when developing platform-independent binaries like those for embedded systems.
Selection of third-party libraries
A language’s versatility is often determined by the third-party support it receives. Cargo, Rust’s official library repository, hosts over 60,000 “crates.” Many of these are API bindings for popular libraries or frameworks. Yet, there isn’t a comprehensive ranking system based on quality for these crates, so you have to rely on your personal experience or community advice.
Find out more about popular open-source Rust libraries in our earlier post.
IDE support
The Rust team developed rust-analyzer to offer real-time feedback from the Rust compiler to IDEs, for example, Microsoft Visual Studio Code.
Open-source nature
As an open-source language, Rust benefits from the contributions of an extensive global developer community that continuously improves its performance. Friendly, especially for those new to programming. It also regularly updates its detailed and transparent language documentation.
The Rust development ecosystem
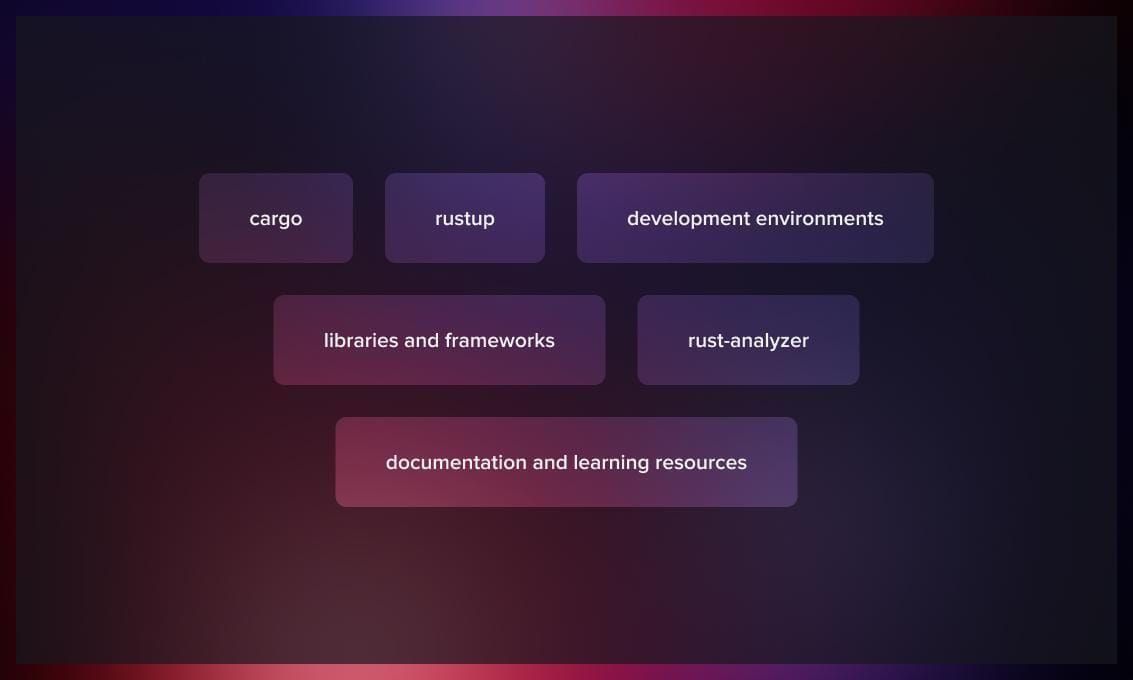
One of Rust’s significant advantages is its wide ecosystem of tools, frameworks, and libraries.
Cargo
Cargo is the official package manager and compiler for Rust. It handles project building, dependency management, and test execution. For the official registry of Rust packages, developers use crates.io, which is governed by the Rust community and is integrated with Cargo.
Rustup
A utility to streamline the management of Rust versions, Rustup allows for switching between different Rust toolchains and updating them.
Development environments
Many IDEs and text editors now support Rust development, including Visual Studio Code, Atom, and IntelliJ IDEA.
Libraries and frameworks
Rust’s library and framework ecosystem is constantly expanding and encompasses applications such as web development, gaming, and machine learning, among others. Among its popular libraries and frameworks are Rocket, Tokio, Serde, and Actix.
Rust-analyzer
An efficient and dependable language server, rust-analyzer offers functionalities like code analysis and auto-completion for Rust code. It is easy to use and compatible with multiple text editors and IDEs.
Rustfmt is a dedicated formatting tool to ensure the uniformity of Rust code.
Documentation and learning resources
Rust provides a lot of useful documentation. Check out some useful resources that we’ve collected here for your reference.
- The Rust Programming Language Book (in-depth explanation of Rust and its core concepts)
- Rust Language Cheat Sheet
- Rust Atomics and Locks. Low-Level Concurrency in Practice by Mara Bos (book)
- Programming Rust: Fast, Safe Systems Development by by Jim Blandy, Jason Orendorff, Leonora Tindall (book)
- Rust by Example (collection of annotated code samples)
- Rust Install documents
- Rust Installer download page
- Rust design patterns
- Rust in Motion (paid video course)
- Rust in blockchain (weekly newsletter)
- Serokell’s selection of the best Rust learning resources
Whether you are starting out with Rust or looking for answers to specific questions related to your projects, Rust’s official website is always an excellent source of perfectly structured information.
Rust development challenges
The Rust programming language offers numerous advantages, such as enhanced security, superior performance, and streamlined concurrent programming, along with ensured memory safety. Nevertheless, Rust isn’t without its flaws. Some of the commonly cited challenges when working with Rust include:
Steep learning curve: Rust’s ownership, borrowing, and lifetimes can be confusing for newcomers, especially those coming from languages without these concepts.
Verbose syntax: The language is sometimes critiqued for its verbose syntax, which can be seen as both a pro (explicit over implicit) and a con (lengthy code for simple tasks).
Limited libraries: While the Rust ecosystem is rapidly growing, it still lags behind more mature languages like Python, Java, or JavaScript in terms of the sheer number and diversity of third-party libraries available.
Compilation times: One of the often-cited drawbacks is longer compilation times. This can slow down development, especially in larger projects.
Maturity: While Rust is a stable language, it’s still younger than many of its competitors. As a result, certain features and best practices are still evolving.
Minimal runtime: This is both a strength and a challenge. While it allows Rust to be used in systems programming and places with constrained resources, it also means that the language doesn’t come with as many built-in features as languages with more extensive runtimes.
Rust vs. C++
Below we introduce a comparison between Rust and C++ provided by Serokell’s site reliability engineer Richard Brežák.
What makes Rust stand out from other languages is its type system, library functions that lean towards the functional paradigm, and its ownership system.
Modern C++ has many features and, in fact, implements much of Rust’s feature set. C++20 forgoes the traditional header/source file split and adopts a modern module system. It also introduces functional aspects like lambdas, maps, folds and even std::optional, which behaves like Option.
Some of these modern C++ features can be a bit clunky to use, but they do exist and work. There is even talk within the C++ developer community of introducing features like proper pattern matching and lifetimes, similar to those in the Rust language, into future C++ standards.
Commonly it’s said that the memory management models of C++ and Rust differ. While it’s partially true that both rely on destructors to deallocate resources, the key distinction lies in the fact that Rust is smarter and stricter in tracking the lifetime of values, enabling it to maintain a reference’s relationship with a value. In contrast, C++ doesn’t preserve this relationship after creating a reference; it essentially only creates a faster copy.
The problems with C++ arise from the necessity of ensuring that only the most recent programming paradigms and features are used. This complicates development and adds mental overhead. In contrast, Rust doesn’t allow you to write subpar code. In some cases, C++’s lenience can be a benefit, especially when interfacing with legacy codebases or quickly prototyping a new program. In the end, C++ offers numerous advantages: It is a very versatile language that can compile to virtually any platform and runs with minimal overhead. Its issues stem from too many options, which sometimes leads programmers to make incorrect choices.
Where is Rust already used?
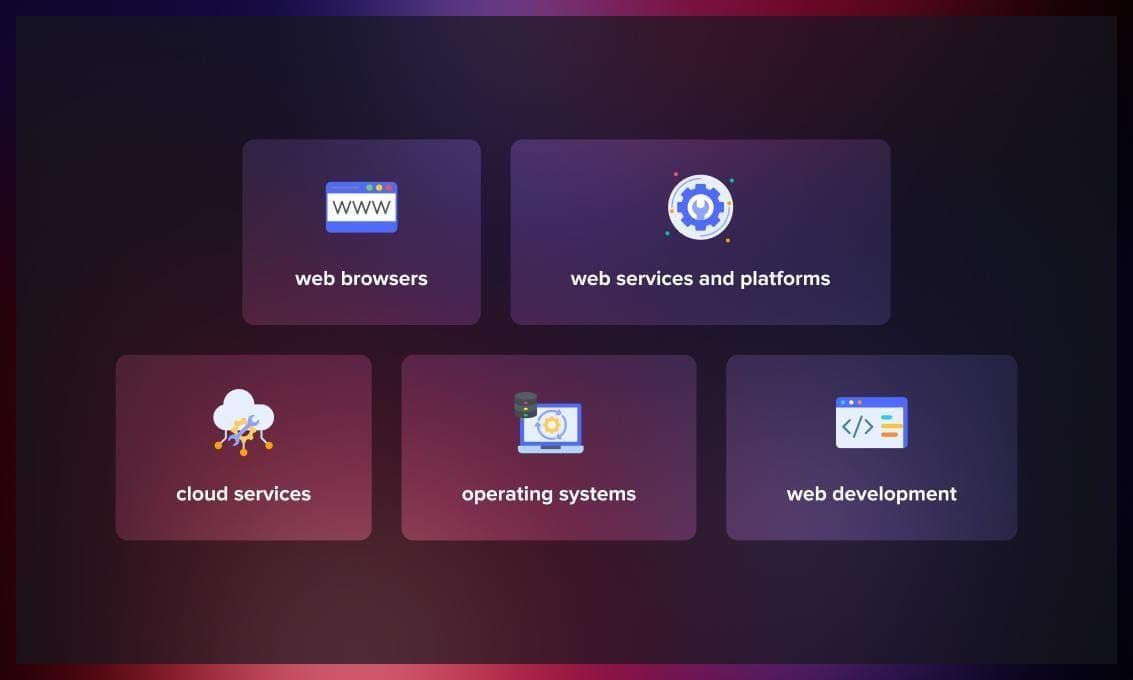
Rust has found multiple applications in various tech domains, which include:
Web browsers
Components from Servo, an experimental parallel browser, were incorporated into Mozilla’s Firefox.
Web services and platforms
Companies like OpenDNS (owned by Cisco) and Cloudflare employ Rust for services like DNS resolution and firewall pattern matching.
- Discord ( an instant messaging social platform) uses Rust for parts of its backend and video encoding.
- Dropbox turned to Rust in 2021 for media capturing.
- Facebook’s (Meta) Mononoke and Google’s Android OS have integrated Rust components.
Cloud services
- Amazon Web Services (AWS) has been using Rust since 2017 for virtualization software (Firecracker), containerization (Bottlerocket), and the asynchronous networking stack (Tokio).
- Microsoft Azure IoT Edge has Rust components. Microsoft also employs them to support containerized modules with WebAssembly and Kubernetes.
Operating systems
- In 2021, the Rust for Linux project aimed to add Rust to the Linux kernel. By 2023, Rust (along with C and Assembly) was incorporated into Linux’s version 6.1.
- Redox OS has a microkernel in Rust.
- Parts of Microsoft Windows were rewritten in Rust for enhanced performance.
Web development
- Deno provides a secure runtime for JavaScript and TypeScript using Rust.
- Ruffle, an open-source SWF emulator, is built in Rust.
To learn more about Rust applications, read our interview series “Rust in Production.”
Conclusion
Surveys show a growing popularity of Rust amongst developers. The 2023 Stack Overflow Developer Survey indicated 13% had recently worked extensively in Rust, with the language being the “most loved” every year from 2016 to 2023. Rust was also ranked 6th in “most wanted technology” in 2023.
The combination of high-level abstractions and granular control make Rust a preferred choice for developing safe and efficient system software. With the increasing recognition of Rust within the developer community it has the potential to become the predominant language in more domains.