Is TypeScript a game-changer in web development? We believe, yes. This statically typed superset of JavaScript is designed to scale up the language, making it more robust and maintainable.
While JavaScript is known for its flexibility, TypeScript brings in the added advantage of type safety, reducing the potential for runtime errors. If you work with JavaScript and want to enhance the reliability of your code and provide more predictable and stable tooling to your customers, TypeScript is the right option.
In this article, we answer the most frequently asked questions about the TS programming language and its use.
How did TypeScript originate?
Around 2012, Microsoft observed the rising complexities of JavaScript applications. The company started to use JavaScript, originally created for simple interactions on web pages, in large-scale applications. Addressing the need for better tooling and scalability, Microsoft introduced TypeScript—a functional programming language that would keep JavaScript’s essence intact while providing tools to build large-scale applications more effectively.
What is TypeScript?
TypeScript is a superset of JavaScript that introduces static typing, interfaces, and other advanced features not present in standard JavaScript.
By incorporating optional static typing, TypeScript allows programmers to catch potential type-related errors at compile-time rather than runtime, enhancing code reliability and maintainability. It provides powerful tools for large-scale application development, including the ability to define custom types, leverage object-oriented programming principles, and use advanced type-checking.
Its integration with modern development tools and IDEs offers improved autocompletion, refactoring, and code navigation capabilities, making the development process more efficient and robust. For the browser to execute TypeScript as it does with JavaScript, TypeScript must be converted into JavaScript. You can use this online compiler for the conversion or utilize the standard compiler provided by Typescript.org.
Why do you need TypeScript?
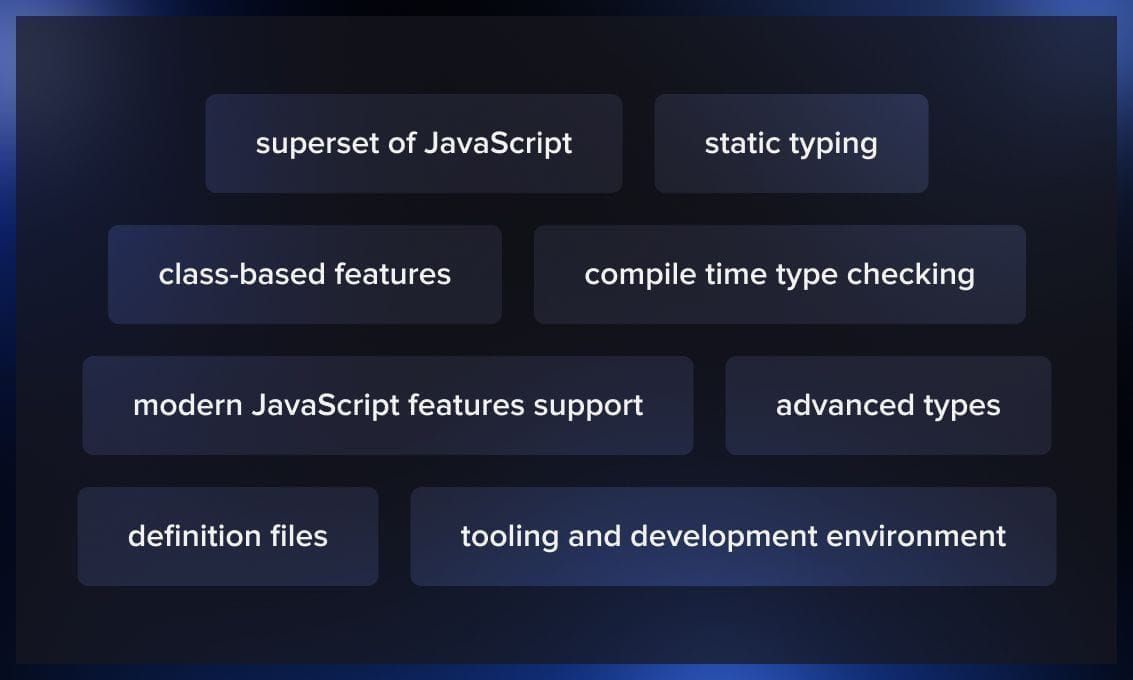
Here are the reasons why you should use TypeScript for your software development projects.
1. Superset of JavaScript
Everything you can do in JavaScript, you can do in TypeScript. It just adds strong typing, and provides special types for JS undefined variables, and some other features to the language.
2. Static typing
One of the main features of TypeScript is static typing. While JavaScript is dynamically typed, in TS you specify the type of a variable and calculate the function according to its mathematical representation. This helps catch a lot of potential errors at compile time, which would otherwise go unnoticed until runtime in the browser.
3. Class-based features
TypeScript adds types to traditional OOP (Object-Oriented Programming) features like classes, interfaces, and namespaces. This makes it easier to manage and organize code, especially for larger projects.
4. Compile time type checking
TypeScript provides a compiler that checks your code for type-related and other errors. When the TypeScript code is compiled, it’s turned into plain JS, which can run in any environment where JavaScript runs.
5. Support for modern JavaScript features
TypeScript is kept up to date with the latest ECMAScript (the specification that JavaScript is based on) features, so you can use modern JS syntax and features, even if you’re targeting older environments, as TypeScript will transpile it to an older version of JavaScript if needed.
6. Advanced types
TS introduces advanced types like union types, intersection types, and type guards. It also provides utilities for type manipulation like Partial
, Pick
, and Omit
.
TypeScript lets developers build new types from existing ones. One of its core features is generics, which makes it easier to reuse types. Plus, TS offers many type operators, which add even more flexibility. By merging different type operators, you can express complex operations and values in a concise and maintainable manner.
7. Definition files
Even if a library is not written in TypeScript, you can use “definition files” (with a .d.ts
extension) to provide type information for that library. This allows you to get the benefits of type checking and autocompletion when using third-party libraries.
8. Tooling and development environment
One of the strengths of TypeScript lies in its development environment and tooling. The TypeScript compiler (tsc
) plays a pivotal role, checking for type errors and transpiling TypeScript code to JavaScript. This provides a safety net, ensuring that type-related errors are caught before the code runs.
Visual Studio Code provides first-class support for TypeScript. This includes features like IntelliSense, which offers auto-completion, refactoring, and inline type-checking, elevating the development experience to new heights.
Typescript vs. JavaScript: What is the difference?
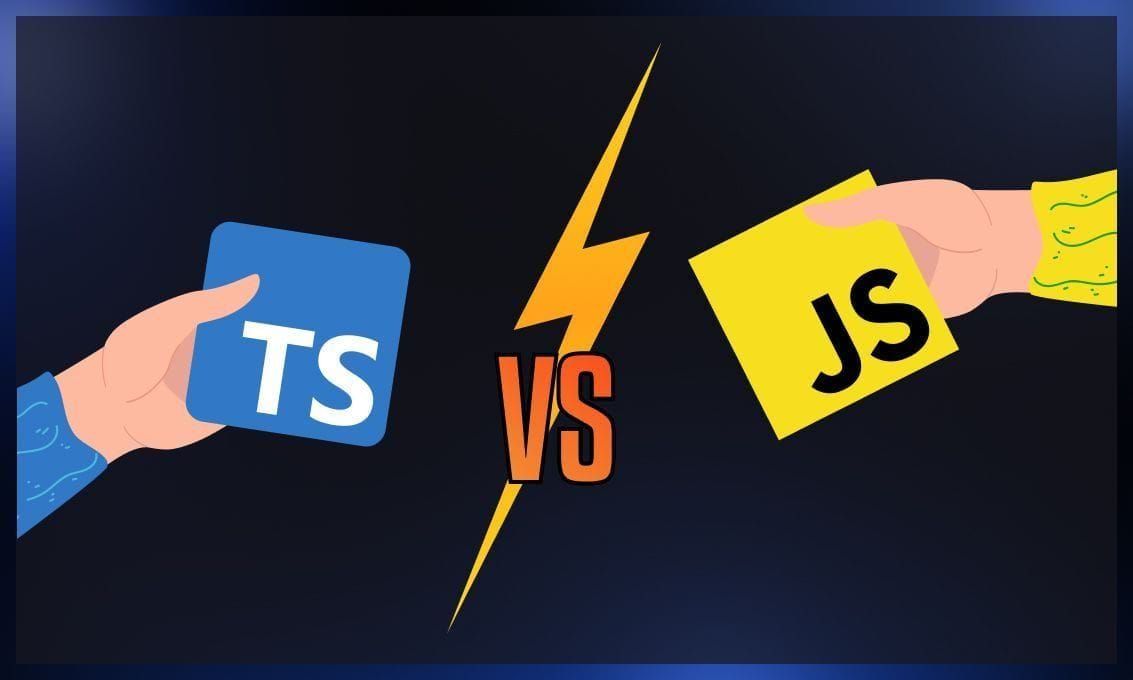
The key differences betweenTypeScript and JavaScript are:
Learning curve
TypeScript is an extension of JavaScript. Therefore, the knowledge of JS is essential for navigating TypeScript. In contrast, JavaScript is known for its user-friendliness. Many developers use JavaScript together with CSS and HTML for designing web applications.
Community support
TypeScript quickly became popular, with many big companies, such as Canva, JPMorgan Chase, Western Union using it. There are lots of online resources and a strong community for TS fans. On the other hand, while JavaScript has many tools and libraries, its community is smaller than that of TypeScript. When deciding between the two, consider your team and what fits best with your company’s goals.
Performance
Since TypeScript was developed to address the challenges of using JavaScript for large and complex applications, it enhances developer productivity and shortens development time. A key difference between TypeScript and JavaScript is the transpilation process: TypeScript is transformed into JavaScript before execution.
Syntax
TypeScript syntax is similar to JScript and .Net, and it fully supports ECMAScript 2015 features like modules, arrow functions, and classes. While JavaScript follows the ECMAScript standard too, it doesn’t have TypeScript’s focus on types. Influenced by C, JavaScript offers structured programming tools such as ‘if’, ‘switch’ statements, and ‘do-while’ loops. It supports various programming styles, including event-driven, functional, and imperative.
Development tools & frameworks
TypeScript works well with modern frameworks and editors, allowing for real-time error checks during compilation. This reduces errors when the code runs. JavaScript, for its part, boasts a variety of frameworks suited for different web development needs. This attracts many developers, including those specializing in popular frameworks like ReactJS, VueJS, and Angular.
Is TypeScript frontend or backend?
TypeScript can be used both in frontend and backend development. It’s all about where you choose to use it.
Frontend: TypeScript is commonly used in frontend development, especially with frameworks and libraries like Angular, React, and Vue. It helps programmers catch type-related errors at compile time, which can lead to more robust and maintainable code in large-scale frontend applications.
Backend: TypeScript can also be used for backend development. For instance, with Node.js, many developers prefer using TypeScript for the added benefits of static typing. Frameworks like NestJS, for example, are built with TypeScript in mind for backend applications.
How to install TypeScript?
Below, you will find useful references for installing TS.
- This quick guide provides essential details on the pre-installation prerequisites, installation steps, and various options you can use.
- If you need a step-by-step tutorial for TS installation on Windows, refer to this tutorial.
- When you encounter difficulties, ask your question on the StackOverflow TS forum or this specific subreddit on Reddit. The proactive community is always ready to assist beginners with detailed explanations, whether they’re related to installation issues or programming challenges.
How to compile TypeScript?
TypeScript, while offering enhanced capabilities over JavaScript, requires an additional step of compilation to produce executable JavaScript code. Read our TypeScript quickstart guide in our earlier TypeScript blog post, where we walk you through the process of effectively compiling TS files.
When do programmers have to use TS?
Functional languages are popular for backend development because they provide a strong mathematical foundation for programming. On the frontend, TypeScript helps catch errors and ensures that data sent between the frontend and backend matches expectations. Together, they make web applications more reliable and easier to manage. Learn more in our blog post “How to Write TypeScript Like a Haskeller.”
How to run typescript in VSСode?
Watch this video to learn how to install and set up TypeScript in Visual Studio Code, transpile and debug your TS program with NodeJS.
How to write and manage SQL server using TS?
Blending the strong typing and structure of TypeScript with SQL server management can lead to more robust and maintainable database applications. However, when you use TypeScript for server code, figuring out how to interact with your database can be tricky. This article offers an in-depth guide on the issue.
Further reading and resources
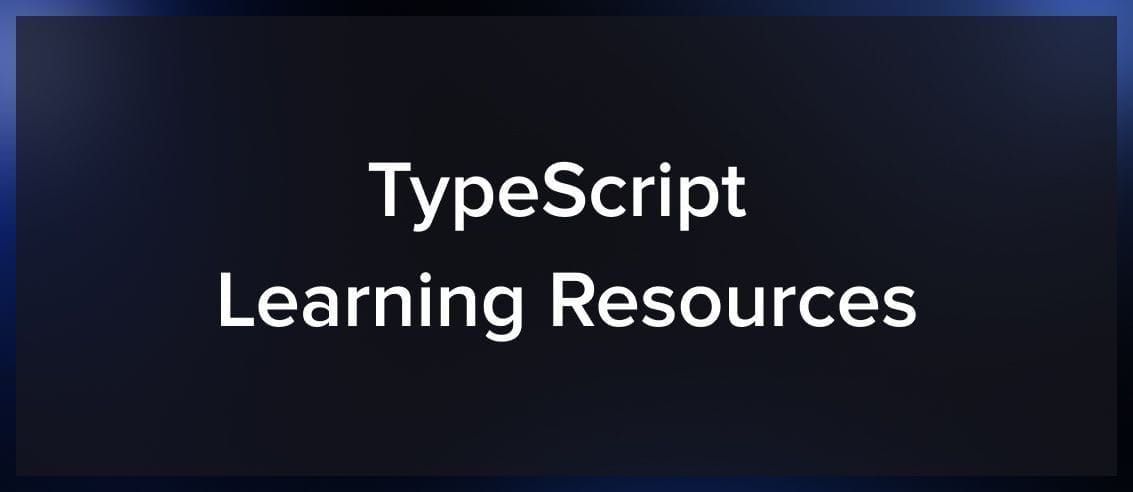
If you’re eager to explore TypeScript further, there’s an abundance of resources available, from official documentation to community-driven tutorials and courses. Here are some useful links:
Tutorials & blogs
- The TypeScript Handbook: The handbook is divided into two main sections. The “TypeScript Handbook” offers a comprehensive guide to TypeScript for regular programmers, explaining its features and behaviors without delving into every minute detail. While it’s not a complete language specification, by the end, readers should understand common TypeScript syntax, the effects of significant compiler options, and be able to predict the type system behavior in most situations. The “Reference Files” section, located below the handbook in the navigation, provides in-depth insights into specific TypeScript concepts without focusing on continuity, allowing readers to gain a deeper understanding of individual topics.
- Official documentation: Here, you’ll find detailed explanations, best practices, and in-depth insights provided by the creators and maintainers of TypeScript.
- TypeScript Evolution: In this blog by Marius Schulz a Front End Engineer at Meta who works on Threads and Instagram, you will find a series of 45 posts on various aspects of TS, such as “Built-In Type Declarations in TypeScript,” “Improved Inference for Literal Types in TypeScript,” “Spelling Correction in TypeScript,” and more.
Books
- Effective TypeScript: 62 Specific Ways to Improve Your TypeScript by Dan Vanderkam: This book offers 62 targeted strategies to refine your TypeScript skills. It is written by Dan Vanderkam, a lead software engineer at Sidewalk Labs. By the end, you’ll go from knowing the basics of TypeScript to understanding its advanced features.
- Programming TypeScript: Making Your JavaScript Applications Scale by Boris Cherny: If you know some JavaScript, author Boris Cherny will show you how to get good at TypeScript. You’ll learn how TypeScript can make your code error-free and easier to manage with more developers.
- Advanced TypeScript Programming Projects by Peter O’Hanlon: This book dives into the latest features of TypeScript 3.0, helping you create powerful apps using frameworks like Angular and React. You’ll learn about TypeScript basics and then build different projects, such as a markdown parser and a photo gallery. The book also touches on advanced topics like Docker with React and machine learning using TensorFlow. By the end, you’ll be skilled in using TypeScript and other JavaScript tools to make great apps. It’s perfect for those who know TypeScript and want to apply it in real situations. No need for experience with other web frameworks.
Tools
- TypeScript Playground: It is an interactive online tool provided by the TypeScript team, allowing developers to experiment with code in real-time. This sandbox environment offers immediate feedback, enabling you to write, test, and view the compiled JavaScript output on-the-fly. Ideal for both beginners seeking to learn the nuances of TypeScript and seasoned developers wanting to test quick snippets, TS Playground simplifies the process of understanding and troubleshooting code.
- TypeScript Error Translator Extension for VS Code: TypeScript errors can be hard to understand. This tool makes them easy to read right in your IDE.
Online сourses
- Understanding TypeScript: This in-depth Udemy course offers a thorough exploration of TypeScript. It spans from basic to advanced features of TypeScript and delves into its integration with ReactJS, Node/Express, and other third-party JavaScript libraries. It also explains TypeScript workflows using webpack and provides ample exercises for practical application. This course is ideal for those seeking a deep understanding of TypeScript, especially Angular learners.
- TypeScript Bootcamp: Zero to Mastery: This ZTM course covers everything from basics to advanced topics. It starts with TypeScript setup, teaches fundamentals like variables and functions, then dives into complex subjects like classes and asynchronous coding. Learners also work on projects like building a weather app and integrating TypeScript in both frontend and backend systems. The course stays updated with the latest TS practices.
Conclusion
TypeScript is an excellent choice for developers aiming to write clean and easily understandable code. Notably, it offers a range of features that can significantly enhance the development process. To have an edge over JavaScript developers, knowledge of TypeScript is definitely an asset for a programmer.
To learn more about TypeScript, read our earlier blog posts: