TypeScript has been increasing in its popularity for the last couple of years. Angular, one of the largest frontend frameworks, is using TypeScript. About 60% of JS programmers already use TypeScript, and 22% wish to try. Why?
Historically, JavaScript has ended up as the main language for scripting web pages and apps on the Internet. It is now possible to use JavaScript on both the frontend and the backend with frameworks like Node.js and Deno.
But was JavaScript made for creating large, complex systems like the ones on the modern web? No.
In this article, we will introduce you to a solution for that – TypeScript – and get you started towards the path of adding types to your JavaScript code.
Serokell has been a long-time provider of Typescript development services, and we’re happy to share our knowledge through this blog.
Here are some of the points we will cover:
- What is TypeScript?
- What are types, and how do they work in TS?
- JavaScript vs. TypeScript: which one to choose?
- How to get started with TypeScript.
- Further resources for studying TypeScript.
What is TypeScript?
In short, TypeScript is a superset of JavaScript that has optional typing and compiles to plain JavaScript.
In simpler words, TypeScript technically is JavaScript with static typing, whenever you want to have it.
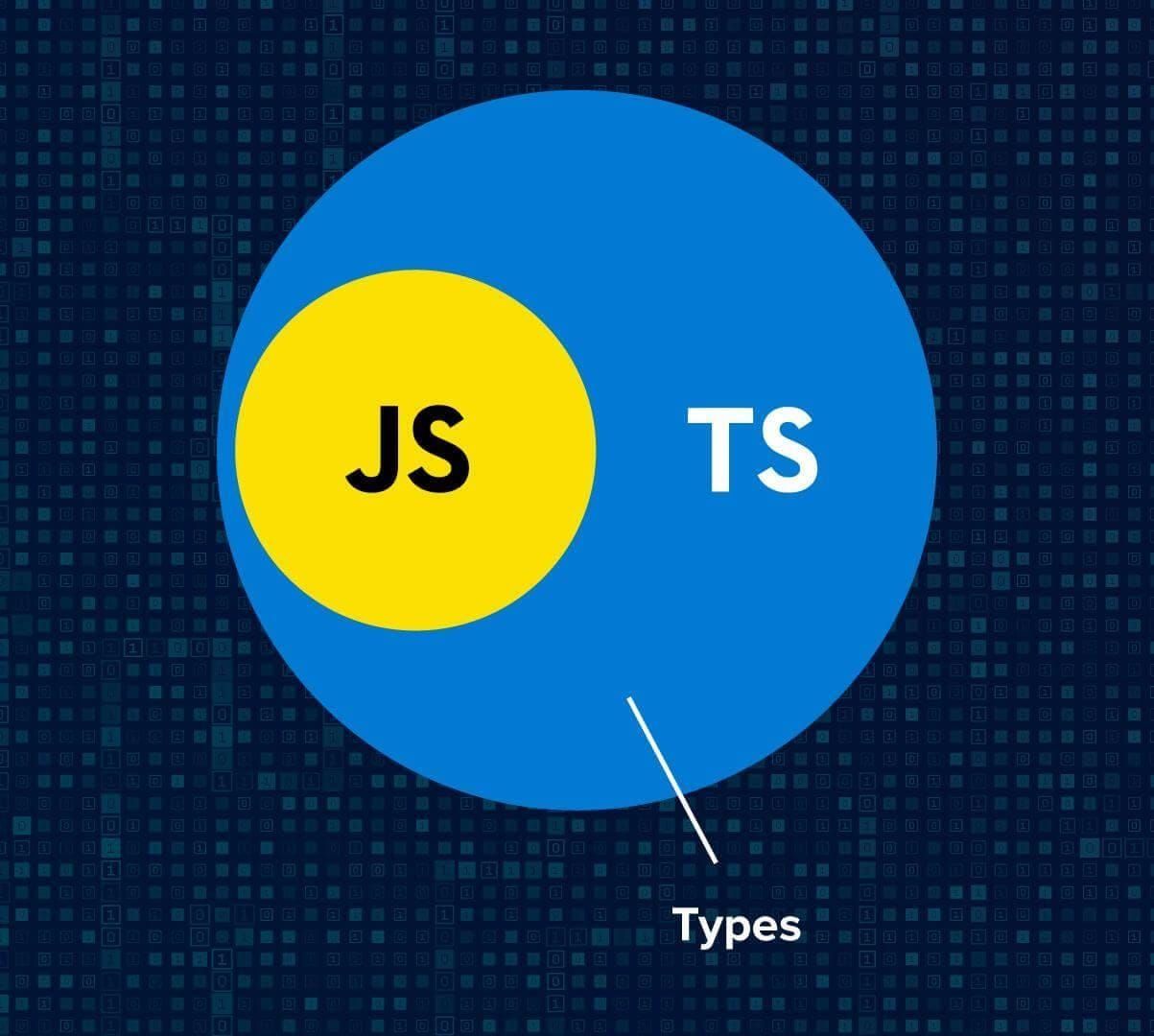
Now, what would be the reasons for adding static typing to JavaScript?
I can list at least three:
- You can avoid masterfully-hidden-ninja errors like the classic
'undefined' is not a function.
- It is easier to refactor code without breaking it significantly.
- Orienting oneself in complex, large-scale systems is not a nightmare anymore.
Actually, a study shows that 15% of all JavaScript bugs can be detected by TypeScript.
The freedom of dynamic typing often leads to bugs that not only decrease the efficiency of the programmer’s work, but can also grind development to halt due to increasing costs of adding new lines of code.
Therefore, the failure of JavaScript to incorporate things like types and compile-time error checks makes it a bad choice for server-side code in enterprises and large codebases. As their tagline says, TypeScript is JavaScript that scales.
What do I need to learn to use TypeScript?
TypeScript is essentially a JS linter. Or, JS with documentation that the compiler can understand.
Therefore, in contrast to other languages like CoffeeScript (which adds syntactic sugar) or PureScript (which does not look like JavaScript at all), you do not need to learn a lot to start writing TypeScript code.
Types in TS are optional, and every JS file is a valid TypeScript file. While the compiler will complain if you have type errors in your initial files, it does give you back a JavaScript file that works as it did before. Wherever you are, TypeScript will meet you there, and it is easy to build up your skills gradually.
Is TypeScript used on the frontend or the backend?
TypeScript is compiled to JavaScript. Therefore, TS can be used anywhere JS could be used: both the frontend and the backend.
JavaScript is the most popular language to implement scripting for the frontend of apps and web pages. Thus, TypeScript can be used for the very same purpose, but it shines in complex enterprise projects on the server side.
At Serokell, most of our web frontend is implemented in TypeScript.
What are types, and how do they work in TS?
Brief intro to types
Types are a way to tell correct programs from incorrect before we run them by describing in our code how we plan to use our data. They can vary from simple types like Number and String to complex structures perfectly modeled for our problem domain.
Programming languages fall into two categories: statically typed or dynamically typed.
In languages with static typing, the type of the variable must be known at compile-time. If we declare a variable, it should be known (or inferrable) by the compiler if it will be a number, a string, or a boolean. Think Java.
In languages with dynamic typing, this is not necessarily so. The type of a variable is known only when running the program. Think Python.
TypeScript can support static typing, while JavaScript doesn’t.
Due to the static typing of TypeScript, you will need to try harder to:
- introduce undefined variables (compile-time warnings help)
- sum two strings that have numbers in them (like “4” + “20” = “420”)
- do operations on things that don’t permit them, such as trimming a number.
With static type systems, you can create your own composite types. This enables engineers to express their intentions in more detail.
Explicit types also make your code self-documenting: they make sure that your variables and functions match what is intended and enable the computer to take care of remembering the surrounding context.
Types of TypeScript
TypeScript has a variety of basic types, like Boolean, Number, String, Array, Tuple, etc. Some of these don’t exist in JS; you can learn more about them in the documentation of TypeScript.
In addition to those, here are some other types we want to feature to showcase the expressivity of TS:
Any & Unknown
While any as a type can cover, well, anything that you wish, unknown is its type-safe counterpart.
Whenever you want to escape the type system, any enables you to assign any JavaScript variable to it. It is frequently used to model incoming variables (from third-party APIs, for example) that have not yet been checked and whose type is unknown.
Unknown is a lot like any, but it won’t let you perform any operations on the variable before it is explicitly type-checked.
Void
Void is used when there is no value returned, for example, as the return type of functions that return nothing.
Never
Never is the return type for something that should never occur, like a function that will throw an exception.
Intersection & Union types
These enable you to create custom types to better fit your logic.
Intersection types enable you to put together several basic types in one type. For example, you could create a custom type Person that has a name: string
and a phone_number: number
. It is equivalent to saying: I want my type to be this and that.
Union types enable for your type to take one of the multiple basic types. For example, you could have a query that returns either result: string
or undefined
. It is equivalent to saying: I want my type to be this or that.
If you think about types as spaces, all of these types quickly make sense.
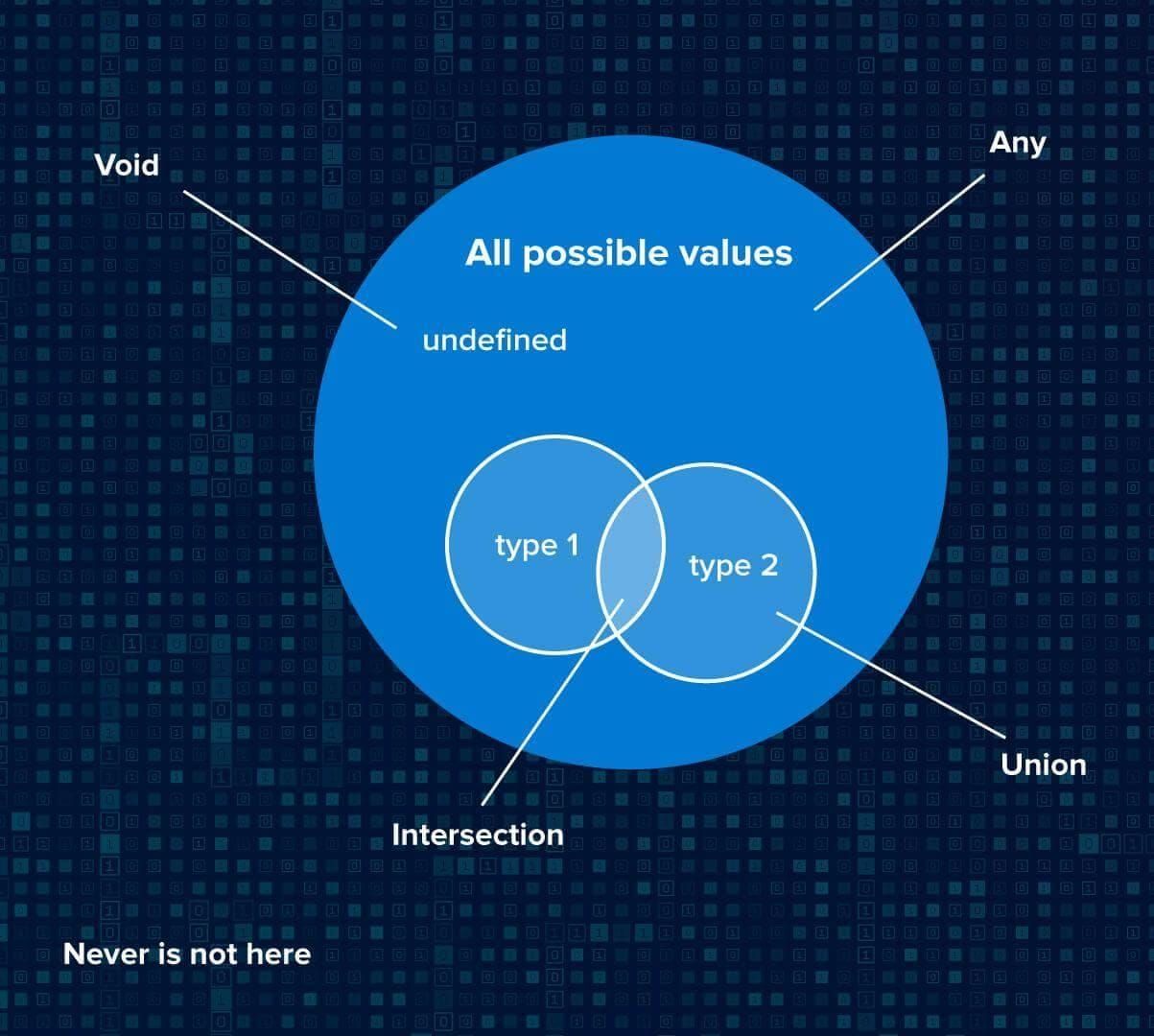
Types in TypeScript can be both implicit and explicit. If you do not explicitly write your types, the compiler will use type inference to infer the types you are using.
Writing them explicitly, however, gives benefits such as helping other developers that read your code and making sure that what you see is what the compiler sees.
TypeScript vs. JavaScript
It pays to be pragmatic. Have a look at this graph:
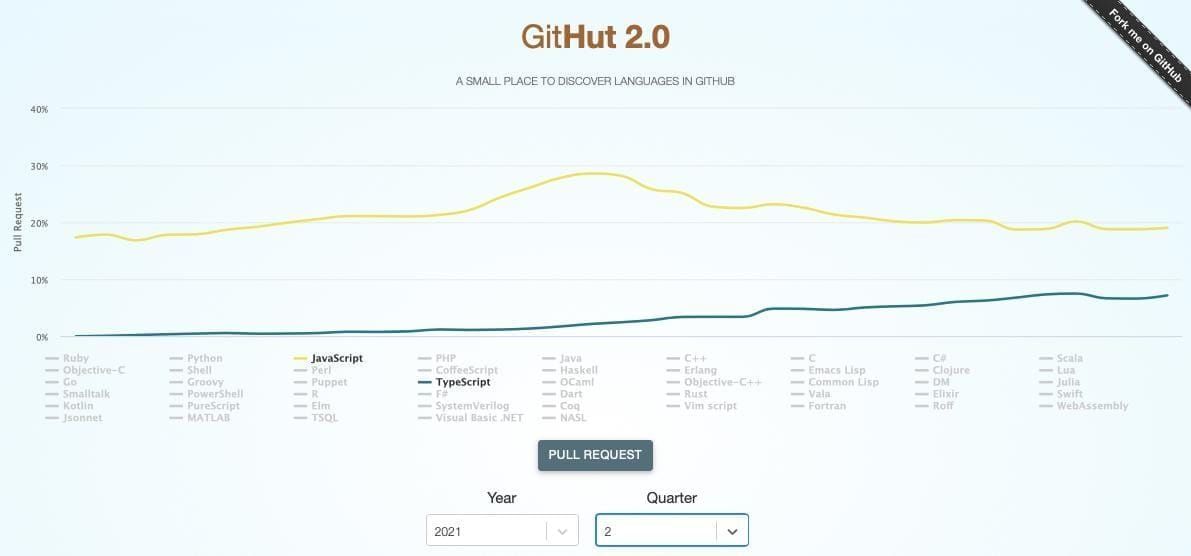
From nowhere, TypeScript is now in the 6th position in GitHub pull requests for Q2 2021, above PHP and C. (Source)
While a considerable cause of this is the support of TypeScript by companies like Microsoft (which created it) and Google, it is supported for a good reason.
3 reasons why you should choose TypeScript over JavaScript
1. TypeScript is more reliable
In contrast to JavaScript, TypeScript code is more reliable and easier to refactor. This enables developers to evade errors and do rewrites much easier.
Types invalidate most of the silly errors that can sneak into JavaScript codebases, and create a quick feedback loop to fix all the little mistakes when writing new code and refactoring.
2. TypeScript is more explicit
Making types explicit focuses our attention on how exactly our system is built, and how different parts of it interact with each other. In large-scale systems, it is important to be able to abstract away the rest of the system while keeping the context in mind. Types enable us to do that.
3. TypeScript and JavaScript are practically interchangeable, so why not?
Since JavaScript is a subset of TypeScript, you can use all JavaScript libraries and code that you want in your TypeScript code.
Most popular JavaScript libraries have types – Definitely Typed is a repository with types for a lot of different JavaScript libraries that you can use to make your interactions with them more type-safe.
This means that you can gradually adopt TypeScript in your JavaScript codebase, first adding types to individual modules and then expanding to… consume the known universe, I guess.
Drawbacks of TypeScript
You can’t just take a JavaScript team or a JavaScript repository and instantly switch them to idiomatic TypeScript. There are tradeoffs, and upfront time sacrifices you have to make.
While we can argue about the savings that being explicit about types give you in the long run, in the short run, it does take more time to add them. This is arguably not a huge deal, but it is an argument in favor of JavaScript.
Therefore, you might not choose TypeScript for small projects and prototypes for your own use.
Tests vs. Types
To briefly touch the discussion of testing vs. types: both of these things catch different classes of bugs, so it makes sense to do both in a nonpartisan-like manner.
You can still use both unit testing and more advanced techniques such as property-based testing with TS while keeping the benefits of a static type system.
To sum it up, here’s a quick comparison of TS and JS languages:
TypeScript | JavaScript |
---|---|
TS is an object-oriented scripting language | JS is an object-oriented scripting language |
Dependent language (compiles to JavaScript) | Independent language (can be interpreted and executed) |
Compiled language, cannot be directly executed in a browser | Interpreted language, executed directly in a web browser |
Can be statically typed | Dynamically typed |
Better structured and concise | More flexible since you are not limited by the type system |
Has a .ts extension | Has a .js extension |
Created at Microsoft by Anders Hejlsberg (designer of C#) and maintained by Microsoft | Created by Brendan Eich (Netscape) and maintained by ECMA (European Computer Manufacturers Association). |
A fair choice for complex projects | Good to work with small, simple projects |
TypeScript quickstart guide
TypeScript compiler
To compile your TS code, you need to install tsc
(short for TypeScript compiler). The easiest way to do it is through the terminal. This can be done easily via npm
by using the following command:
npm install -g typescript
If you want to use TypeScript with Visual Studio Code, there is a handy guide on their website.
Once you have installed tsc
, you can compile your files with tsc filename.ts
.
Migrating your files from JavaScript to TypeScript
Let’s say that we want to change the following JavaScript file to TypeScript due to odd behavior:
function my_sum(a, b) {
return a + b;
}
let a = 4;
let b = "5";
my_sum(a, b);
Good news. Any JS file is technically a valid TypeScript file, so you’re up to a great start – just switch the file extension to .ts from .js.
TypeScript has type inference, which means that it can automatically infer some of the types you use without you adding them. In this case, it presumes that the function sums two variables of type any, which is true but of no great use right now.
If we want to sum only numbers, we can add a type signature to my_sum
to make it accept only numbers.
function my_sum(a: number, b: number) {
return a + b;
}
let a = 4;
let b = "5";
my_sum(a, b);
Now, TypeScript provides us with an error.
Argument of type 'string' is not assignable to parameter of type 'number'.
Good thing we found where the error is. :) To further escape errors like these, you can also add type definitions to variables.
let b: number = "5" // Type '"5"' is not assignable to type 'number'.
let b: number = 5 // Everything ok.
TypeScript is quite flexible in what it can do and how it can help you. For a less trivial example on how to move your existing JavaScript codebase to TypeScript or use TypeScript to improve your JS code, read this guide.
How to use TypeScript in a browser?
To run TypeScript in a browser, it needs to be transpiled into JavaScript with the TypeScript compiler (tsc). In this case, tsc
creates a new .js file based on the .ts code, which you can use any way you could use a JavaScript file.
Resources for further learning
If you want to learn more about TypeScript, here are a few other resources that you can check out:
-
TypeScript Documentation. The official documentation features great guides for beginner TypeScripters, including guides for those switching from other programming languages.
-
TypeScript Deep Dive. This free web resource has everything you need to start off with TypeScript, including more detailed explanations of the sections we’ve already covered here.
-
Programming TypeScript: Making Your JavaScript Applications Scale. This is a great book for those of you already fluent in JavaScript. It will help you get up and running with writing TypeScript in no time.
-
Learn TypeScript From Scratch! A practical 3-hour course that goes through all the basic functions of TypeScript, how to use it to interact with some JS frameworks, and how to use the power of TypeScript while writing JavaScript.
-
Exercism. This is the best resource to practice writing code in a new programming language. Mentors that will steer you towards idiomatic code, lots of fun practice tasks – there is nothing not to love about this website.
Conclusions
Overall, TypeScript is a great tool to have in your toolset even if you don’t use it to its full capacity. It’s easy to start small and grow slowly, learning and adding new features as you go. TypeScript is pragmatic and welcoming to beginners, so there is no need to be afraid. I hope this post will be useful in your TypeScript journey. If you want help or have some questions, be sure to ask them on our social media like Twitter or Facebook.
The article was last modified on September 15th, 2021.