How to Preprocess Data in Python
Before training a model, you have to preprocess data. This is necessary to transform raw data into clean data suitable for analysis.
In this guide, we will cover essential steps to preprocess data using Python. These include splitting the dataset into training and validation sets, handling missing values, managing categorical features, and normalizing the dataset.
Why do you need to preprocess data?
Data preprocessing is important for several reasons:
- Improves data quality. Data preprocessing techniques such as handling missing values, removing outliers, and correcting inconsistencies help improve the quality and reliability of the data. By ensuring data integrity, we can build more accurate models.
- Enhances model performance. Data preprocessing techniques allow us to handle various challenges present in real-world datasets, such as noise, imbalance, and irrelevant features. By addressing these issues, we can improve the performance and generalization capabilities of our machine learning models.
- Enables feature extraction. Preprocessing techniques, such as dimensionality reduction and feature scaling, enable us to extract relevant information from the dataset. This helps in reducing computational complexity, improving interpretability, and identifying the most influential features for model training.
- Facilitates compatibility. Different algorithms have different requirements regarding the input data format. Preprocessing ensures that the data is in a compatible format for the chosen algorithm, enabling seamless integration and accurate results.
- Increases efficiency. It can reduce the computational time both in training and in the deployed model itself.
Only after your data has been properly preprocessed can you start training your model.
What happens if you don’t preprocess data?
If you choose to skip preprocessing your models, you can face several challenges:
- Inaccurate and unreliable models. If we neglect data preprocessing, our models may suffer from poor accuracy and reliability. Unprocessed data may contain missing values, outliers, or inconsistent formats, leading to biased or incorrect predictions.
- Overfitting or underfitting. Without proper preprocessing, our models may become overly complex or too simplistic. This can result in overfitting, where the model memorizes the noise in the data instead of learning meaningful patterns, or underfitting, where the model fails to capture important relationships due to oversimplification.
- Inefficient resource utilization. Unprocessed data often contains redundant or irrelevant features, leading to increased computational complexity and longer training times. Preprocessing helps eliminate these redundancies, making the model more efficient and reducing resource utilization.
- Biased or unfair results. Unprocessed data may contain biases or skewed distributions, leading to biased or unfair predictions. Data preprocessing techniques can help mitigate these biases, ensuring fairness and ethical considerations in machine learning applications.
Now let us discuss how to preprocess data step by step.
The steps of preprocessing data in Python
Preprocessing in Python happens in several steps.
Step 1: Splitting the dataset into training and validation sets
Splitting the dataset into training and validation sets is crucial for evaluating model performance, preventing overfitting, tuning hyperparameters, assessing generalization capabilities, and avoiding data leakage.
This is what you need to do:
1. Import the necessary libraries
import pandas as pd
from sklearn.model_selection import train_test_split
2. Load the dataset
data = pd.read_csv('your_dataset.csv')
3. Split the dataset
train_data, val_data = train_test_split(data, test_size=0.2, random_state=42)
Here, test_size
determines the proportion of the dataset allocated for validation. Adjust it according to your requirements.
Step 2: Handling missing values
Handling missing values in data preprocessing is essential for accurate analysis, avoiding biased results, preserving data integrity, maintaining model performance, and preventing errors. It ensures that our data is complete and reliable, leading to more robust and meaningful insights and predictions.
Consider these approaches to handle missing values:
1. Identify missing values
missing_values = data.isnull().sum()
2. Decide on handling missing values based on the context
- Delete rows with missing values:
data = data.dropna()
-
Fill missing values with mean/median/mode:
data['column_name'].fillna(data['column_name'].mean(), inplace=True)
-
Use advanced imputation techniques (e.g., K-Nearest Neighbors) if applicable.
Step 3: Managing categorical features
Managing categorical features in machine learning refers to the process of transforming or encoding categorical variables in a way that can be effectively used by the model. Categorical features are variables that represent qualitative or non-numeric data, such as gender, color, or country.
Here are some common techniques for managing categorical features:
One-hot encoding
This technique converts each category in a feature into a binary column. For example, if a feature has three categories (red, green, blue), it will be transformed into three binary columns (red: 1 or 0, green: 1 or 0, blue: 1 or 0). One-hot encoding allows the model to understand the categorical feature without assuming any ordinal relationship between the categories.
Label encoding
Label encoding assigns a unique numerical value to each category in a feature. For example, if a feature has three categories (red, green, blue), they can be encoded as (0, 1, 2). However, label encoding assumes an ordinal relationship between the categories, which may not always be appropriate.
Ordinal encoding
Ordinal encoding is similar to label encoding but preserves the order of the categories. It assigns numerical values based on the order of the categories. For example, if a feature has three categories (low, medium, high), they can be encoded as (0, 1, 2). Ordinal encoding is useful when there is a clear ordering or hierarchy among the categories.
Count encoding
Count encoding replaces each category with the count of its occurrences in the dataset. This can be useful when the frequency of a category is informative and can help the model make predictions.
Target encoding
Target encoding replaces each category with the mean target value of the corresponding category. This technique is useful when there is a relationship between the target variable and the categorical feature.
The choice of categorical feature management technique depends on the nature of the data, the specific problem, and the machine learning algorithm being used.
Consider these steps:
1. Identify categorical features
categorical_features = data.select_dtypes(include=['object']).columns
2. Convert categorical features into numerical representations
-
One-hot encoding:
encoded_data = pd.get_dummies(data, columns=categorical_features)
-
Label encoding:
from sklearn.preprocessing import LabelEncoder label_encoder = LabelEncoder() for feature in categorical_features: data[feature] = label_encoder.fit_transform(data[feature])
Step 4: Normalization of the dataset
Normalizing the dataset ensures that all features have a consistent scale, preventing any particular feature from dominating the model.
Normalization of a dataset refers to the process of scaling the values of numerical features to a standard range, typically between 0 and 1 or -1 and 1. It is necessary for several reasons:
- Preventing feature dominance. If the numerical features have different scales or units, some features may dominate others in terms of their magnitude. This can lead to biased results and inaccurate model predictions. Normalization ensures that all features contribute equally to the model’s learning process.
- Improving convergence. Many machine learning algorithms rely on optimization techniques that converge faster when the features are within a similar scale. Normalization helps in achieving faster convergence, which can lead to more efficient training and better model performance.
- Handling outliers. Outliers are extreme values that can disproportionately influence the model’s learning process. Normalization can help in reducing the impact of outliers by bringing all values within a standardized range.
- Facilitating interpretation. Normalization makes it easier to compare and interpret the coefficients or feature importance values obtained from the model. When features are on different scales, it becomes challenging to determine the relative importance of each feature.
There are different methods for normalization, such as Min-Max scaling, Z-score normalization, and robust scaling. The choice of normalization method depends on the specific requirements of the dataset and the machine learning algorithm being used.
Follow these steps:
1. Import the necessary libraries
from sklearn.preprocessing import MinMaxScaler
2. Normalize the dataset
scaler = MinMaxScaler()
normalized_data = scaler.fit_transform(data)
Note: Normalization is not always necessary, especially for certain algorithms like decision trees or random forests.
Conclusion
Data preprocessing is a critical step in machine learning projects that cannot be overlooked. It ensures data quality, enhances model performance, enables feature extraction, and facilitates compatibility with different algorithms. Neglecting data preprocessing can lead to inaccurate models, overfitting or underfitting, inefficient resource utilization, and biased results. Therefore, it is essential to invest time and effort in preprocessing data to achieve robust and accurate machine learning models.
Further reading:
- Why do you need to preprocess data?
- The steps of preprocessing data in Python
- Conclusion
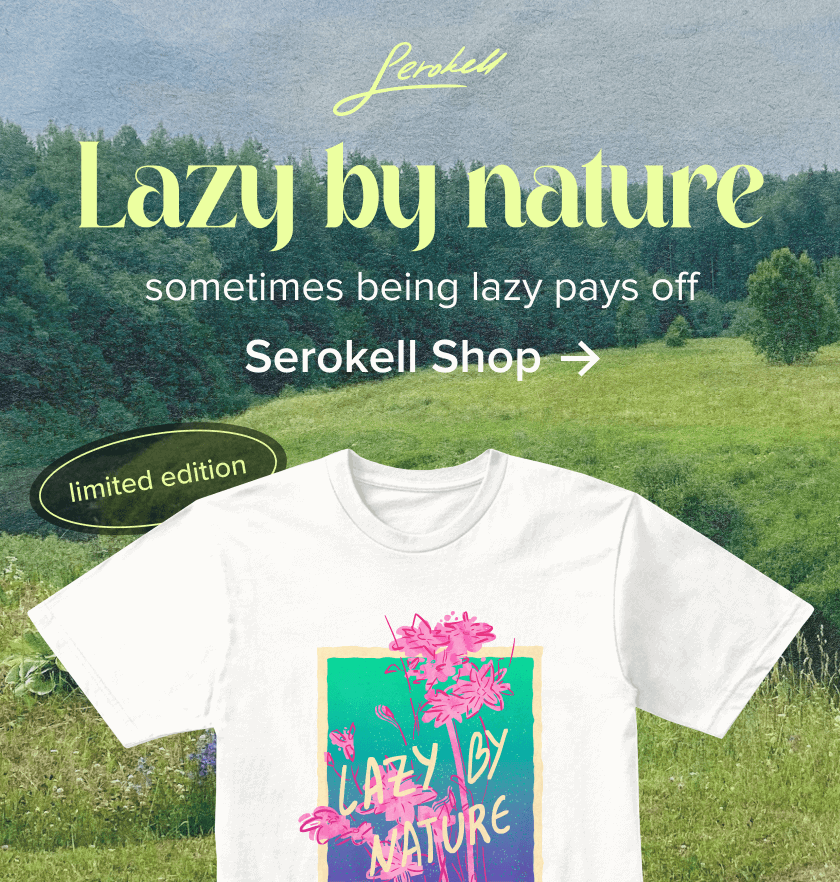
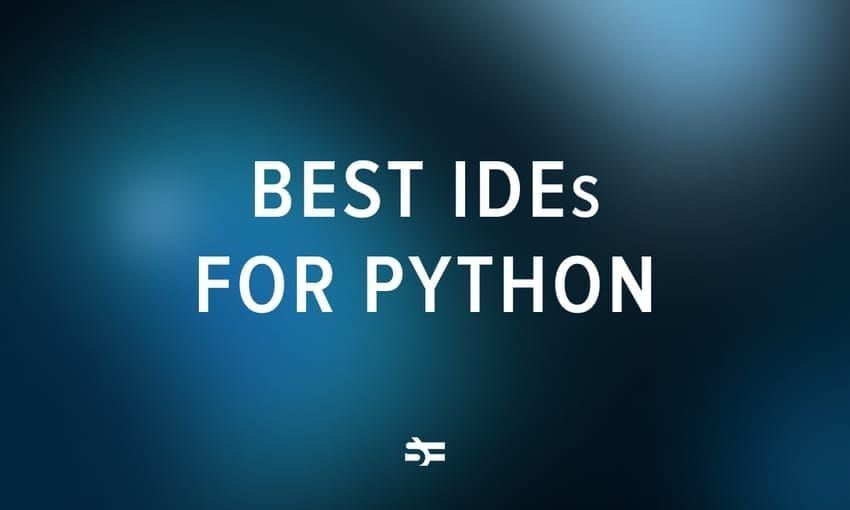
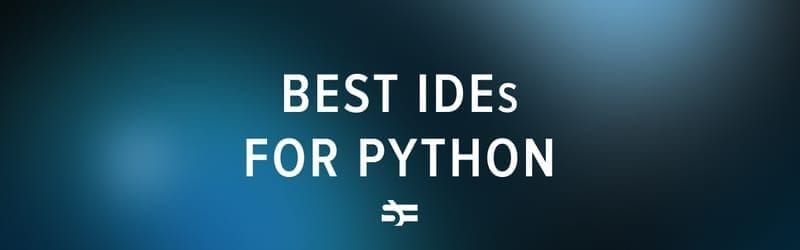
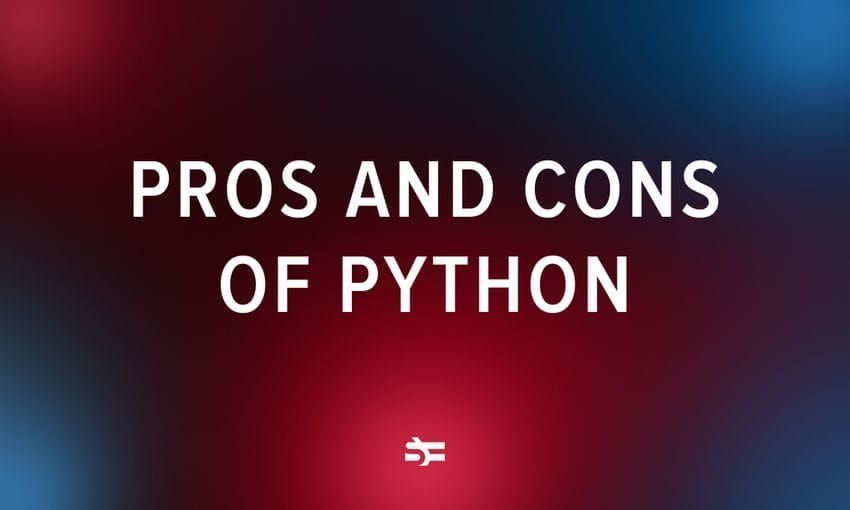
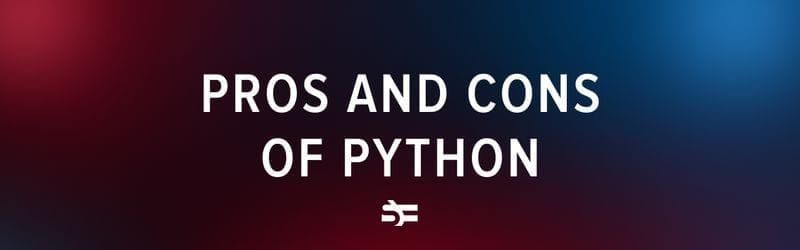
.jpg)
.jpg)